… as a project dependency.
A problem I came across recently when working on a project with a colleague was setting up WordPress Coding Standards (WPCS) with PHP Code Sniffer (PHPCS).
I already had it setup on my development machine, with PHPCS installed globally as well as the WP Coding Standards. But lets say we want to encourage use of WPCS in a project where we might have different team members involved, then I think its a good idea to install it as a dependency.
What are the WP Coding Standards, and what is PHPCS
- The WP Coding Standards are a set of guidlines to follow when building a plugin or theme on top of WordPress. In this post I’m referring to the PHP Coding standards.
- PHPCS – is a command line tool to detect issues in your PHP code by comparing it against a set of rules that you can define. In this case the WordPress coding standards rules. You can also configure it to format your PHP files for you.
To get PHPCS to understand and apply the WPCS rules to your code, there is the WordPress Coding Standards PHPCS repository which we’ll be installing via Composer into your project, it comes with a copy of PHPCS.
Prerequisites
This post assumes you already have Composer setup on your machine and that you’re familiar with creating repositories and adding composer to your project via the command line.
I’m also writing this from a Windows machine, so you might need to fiddle with paths etc if you’re using something else – double check the installation paths of your packages when using Composer.
Setup
Add the package to your project
If you haven’t setup composer in your project yet, at the command line run:
composer init
And follow the usual prompts to configure your project.
Then require WordPress Coding Standards which will also give you a local copy of PHPCS, run:
composer require wp-coding-standards/wpcs
Add WPCS rules location to PHPCS
Once that is installed we need to tell PHPCS the location of our WordPress rules, we can run:
vendor/bin/phpcs --config-set installed_paths ../../wp-coding-standards/wpcs
Note: I’m using a relative path of the rules to the PHPCS installation ../../wp-coding-standards/wpcs
. You can use an absolute path too.
Automating this for developers
When developers setup your repository and install the projects dependencies via composer install
it might be nice to simplify the above step by running it automatically after we run the install – via a post install script.
This requires adding a “scripts” section after your “require” section in composer.json
, so this:
"require": {
"wp-coding-standards/wpcs": "^2.3"
},
Would become this:
"require": {
"wp-coding-standards/wpcs": "^2.3"
},
"scripts": {
"post-update-cmd": [
"vendor/bin/phpcs --config-set installed_paths ../../wp-coding-standards/wpcs"
]
}
Once you’ve added that, you can be sure the next team members on your project will automatically get PHPCS configured with WPCS out of the box.
Configure your IDE (VS Code)
If you’re using VS Code, I would recommend the PHP Sniffer extension by wongjn which allows us to format the code on save too.
Once installed, open the extensions settings and take note to switch the workspace tab.
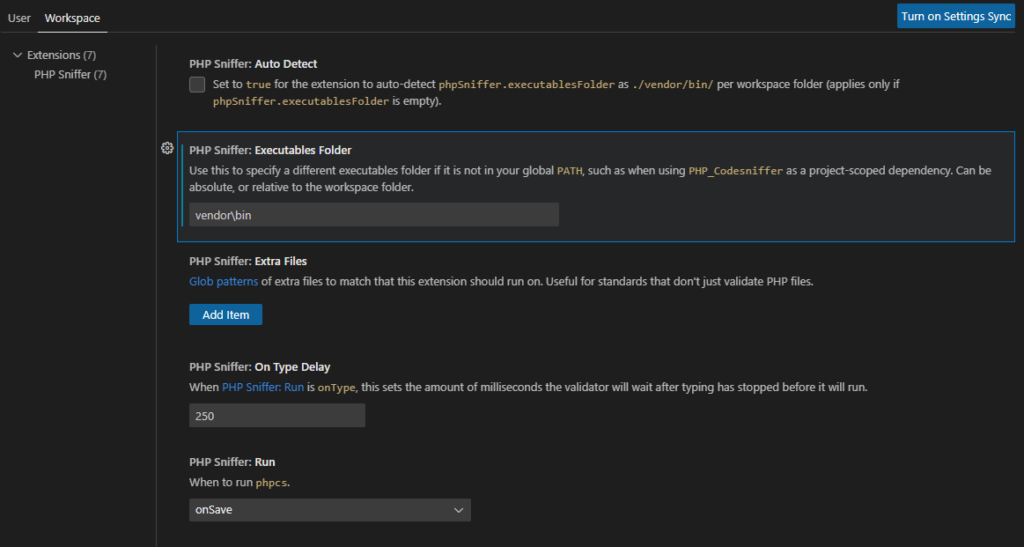
I was happy with the defaults, the only thing we need to do is add our executable folder as vendor\bin
.
If you check your workspace settings, it should look like:
{
"phpSniffer.executablesFolder": "vendor\\bin",
}
Note: instead of setting a path, you could let PHP Sniffer try to autodetect this for you:

Personally, I like the re-assurance of specifying the location.
Auto formatting on save (phpcbf)
To tell VS Code we want to format on save, we just need to include the editor.formatOnSave
directive in our workspace settings:
{
"phpSniffer.executablesFolder": "vendor\\bin",
"editor.formatOnSave": true,
"[php]": {
"editor.defaultFormatter": "wongjn.php-sniffer"
},
}
Depending on your setup, you might also want to tell VSCode to use the extension specifically for formatting php files using the [php]
property and then defining editor.defaultFormatter
.
Configure the WordPress Coding Standards
We can configure the extension to use the WordPress rules directly or (which I prefer), we can instead create custom rules based on the WPCS rules which will allow us to customise them at a later date – we’ll call our custom ruleset RmCodesRuleSet
.
To do this we can create a .phpcs.xml
file in the root of our project with the contents:
<?xml version="1.0"?>
<ruleset name="RmCodesRuleSet">
<rule ref="WordPress"/>
</ruleset>
The phpcs extension will automatically read this file and the <rule ref="WordPress">
so it knows to inherit the WordPress Coding Standard Rules.
The VS Code PHP Sniffer extension (as well as many others) will usually load a .phpcs.xml
file automatically.
Excluding some rules
Finally, if you want to exclude specific rules we can do that by using the exclude <exclude>
tags.
I often exclude the filename restrictions as I’m usually using namespaces these days (with a different file structure) and the yoda conditions I’ve heard will be removed from the coding standards at some point anyway.
<?xml version="1.0"?>
<ruleset name="RmCodesRuleSet">
<rule ref="WordPress">
<!-- Exclude the filename restrictions assocatied with class name -->
<exclude name="WordPress.Files.FileName"/>
<!-- Exclude the requirement to use Yoda conditions -->
<exclude name="WordPress.PHP.YodaConditions.NotYoda"/>
</rule>
</ruleset>
Taking it further
Aside from setting up PHPCS + WPCS in your repository and IDE, we could also configure it to run on push via Github actions.
But that’ll probably be another article…